Introduction#
The Solver API (SAPI) is an interface to D‑Wave quantum computers and hybrid and classical solvers in the Leap service. This document describes the SAPI interface, including the calls you may use to submit and manage problems and access the available solvers.
A REST-based web services API, SAPI is fairly simple to use; it exposes only a few basic calls. Most of the work for you will be restructuring your problem into a format that the API can answer, and then parsing results.
Note
D‑Wave provides a full-stack open-source Software Development Kit (SDK), Ocean SDK. It is recommended that you develop your applications on top of that.
This document is intended for developers who want to build their own applications on top of the SAPI REST interface. Readers should be familiar with the HTTP protocol.
HTTP Response Codes#
You can see a list of HTTP response codes here. The following table describes some of the most frequently encountered codes.
Code |
Explanation |
---|---|
200 OK |
No error. |
201 CREATED |
Creation of a resource was successful. |
202 ACCEPTED |
Request such as problem cancellation was received. |
304 NOT MODIFIED |
The requested resource has not changed since
the time specified in the request’s
|
400 BAD REQUEST |
Invalid request URI or header, or unsupported nonstandard parameter. |
401 UNAUTHORIZED |
Authorization required. This error can also mean that incorrect user credentials were sent with the request. |
403 FORBIDDEN |
Unsupported standard parameter, or authentication or authorization failed. |
404 NOT FOUND |
Resource (such as a problem) not found. |
409 CONFLICT |
Conflict such as a request for problem cancellation for a problem that has already terminated. |
429 TOO MANY REQUESTS |
API request rate exceeds the permissible limit. |
500 INTERNAL SERVER ERROR |
Internal error. This is the default code that is used for all unrecognized server errors. |
SAPI Problem Lifecycle#
A problem may have one of the following statuses:
Status |
Description |
---|---|
PENDING |
Problem was submitted and is queued for processing. |
IN_PROGRESS |
Problem is currently being processed. |
COMPLETED |
Problem completed successfully. |
FAILED |
Problem processing failed. |
CANCELLED |
Problem was cancelled. |
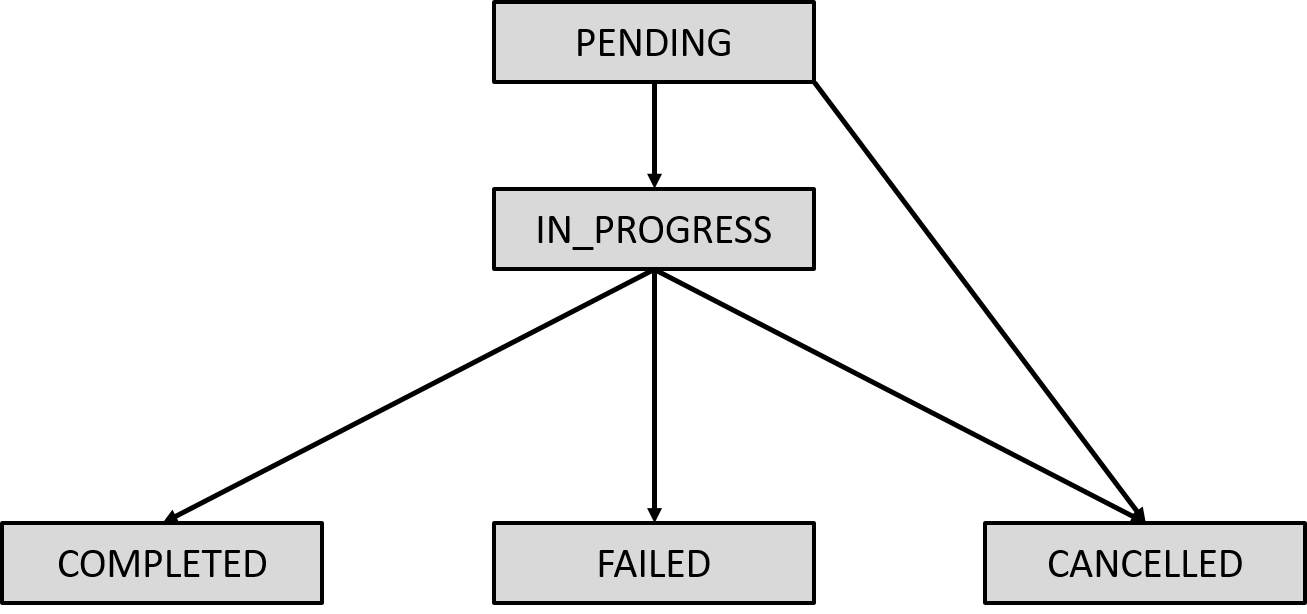
Fig. 129 Initially a problem is in the PENDING state. When a solver starts to process a problem, its state changes to IN_PROGRESS. After completion, the problem status changes to either COMPLETED or FAILED (if an error occurred). COMPLETED, FAILED, and CANCELLED are all terminal states. After a problem enters a terminal state, its status does not change. Users can cancel a problem at any time before it reaches its terminal state.#
SAPI Version#
To ensure compatibility, verify the version of the SAPI resource you are using in your code. You can see the version number in the response header sent from SAPI.
The base URL and authorization token used in the following example are explained in the Set Up the Session section.
>>> import requests
...
>>> SAPI_HOME = "https://na-west-1.cloud.dwavesys.com/sapi/v2"
... # Replace with your API token
>>> SAPI_TOKEN = "ABC-1234567...345678"
...
>>> r = requests.get(f"{SAPI_HOME}/problems/?max_results=3", headers={'X-Auth-Token': SAPI_TOKEN})
>>> print(r.headers["Content-Type"])
application/vnd.dwave.sapi.problems+json; version=2.1.0; charset=utf-8